Java 并发编程之 AQS CyclicBarrier 源码解析
Java juc AQS About 3,284 words说明
CyclicBarrier
与CountDownLatch
的差别在于:CyclicBarrier
可重复使用,而CountDownLatch
在计数归0
后就不能再用。
await
使用ReentrantLock
和Condition
保证同步和等待。
// java.util.concurrent.CyclicBarrier#await()
public int await() throws InterruptedException, BrokenBarrierException {
try {
return dowait(false, 0L);
} catch (TimeoutException toe) {
throw new Error(toe); // cannot happen
}
}
private int dowait(boolean timed, long nanos) throws InterruptedException, BrokenBarrierException, TimeoutException {
final ReentrantLock lock = this.lock;
lock.lock();
try {
final Generation g = generation;
if (g.broken)
throw new BrokenBarrierException();
if (Thread.interrupted()) {
breakBarrier();
throw new InterruptedException();
}
int index = --count;
if (index == 0) { // tripped
boolean ranAction = false;
try {
final Runnable command = barrierCommand;
if (command != null)
command.run();
ranAction = true;
nextGeneration();
return 0;
} finally {
if (!ranAction)
breakBarrier();
}
}
// loop until tripped, broken, interrupted, or timed out
for (;;) {
try {
if (!timed)
trip.await();
else if (nanos > 0L)
nanos = trip.awaitNanos(nanos);
} catch (InterruptedException ie) {
if (g == generation && ! g.broken) {
breakBarrier();
throw ie;
} else {
// We're about to finish waiting even if we had not
// been interrupted, so this interrupt is deemed to
// "belong" to subsequent execution.
Thread.currentThread().interrupt();
}
}
if (g.broken)
throw new BrokenBarrierException();
if (g != generation)
return index;
if (timed && nanos <= 0L) {
breakBarrier();
throw new TimeoutException();
}
}
} finally {
lock.unlock();
}
}
private void nextGeneration() {
// signal completion of last generation
trip.signalAll();
// set up next generation
count = parties;
generation = new Generation();
}
private void breakBarrier() {
generation.broken = true;
count = parties;
trip.signalAll();
}
nextGeneration
:将计数重置,且唤醒所有await
的线程。
count
为0
时,调用CyclicBarrier
中构造方法传入的Runnable
,Runnable
中有异常未捕获时,会重置计数,且唤醒所有await
线程,并标识已经被break
了,之后再执行await
会抛出BrokenBarrierException
异常。举例:
public class CyclicBarrierRunnableExceptionDemo {
public static void main(String[] args) throws BrokenBarrierException, InterruptedException {
CyclicBarrier cyclicBarrier = null;
try {
cyclicBarrier = new CyclicBarrier(1, () -> {
int i = 1 / 0;
});
cyclicBarrier.await();
} catch (Exception e) {
System.out.println(e);
cyclicBarrier.await();
}
}
}
Views: 1,896 · Posted: 2021-10-13
————        END        ————
Give me a Star, Thanks:)
https://github.com/fendoudebb/LiteNote扫描下方二维码关注公众号和小程序↓↓↓
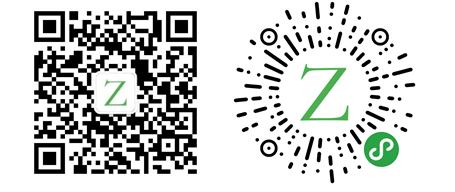
Loading...