设计模式之组合模式
设计模式 Java About 3,841 words作用
又叫部分整体模式,它创建了对象组的树形结构,将对象组合成树状结构以表示“整体-部分”的层次关系,Composite使得用户对单个对象和组合对象的使用具有一致性。
原理
Component:组合中对象的接口,适当情况下实现所有类共有方法的默认行为,用于管理和访问Component子部件,Component可以是抽象类或者接口。
Composite:非叶子节点,用于存储子部件,在Component接口中实现子部件的相关操作,比如增加、删除。
Leaf:在组合中表示叶子节点,叶子节点没有子节点。
案例
组织构件
public abstract class OrganizationComponent {
private String name;
private String desc;
public OrganizationComponent(String name, String desc) {
this.name = name;
this.desc = desc;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getDesc() {
return desc;
}
public void setDesc(String desc) {
this.desc = desc;
}
public void add(OrganizationComponent organizationComponent) {
throw new UnsupportedOperationException();
}
public void remove(OrganizationComponent organizationComponent) {
throw new UnsupportedOperationException();
}
public abstract void print();
}
大学
public class University extends OrganizationComponent {
private List<OrganizationComponent> organizationComponents = new ArrayList<>();
public University(String name, String desc) {
super(name, desc);
}
@Override
public void add(OrganizationComponent organizationComponent) {
this.organizationComponents.add(organizationComponent);
}
@Override
public void remove(OrganizationComponent organizationComponent) {
this.organizationComponents.remove(organizationComponent);
}
@Override
public void print() {
System.out.println("----------" + getName() + "----------");
organizationComponents.forEach(OrganizationComponent::print);
}
}
学院
public class College extends OrganizationComponent {
private List<OrganizationComponent> organizationComponents = new ArrayList<>();
public College(String name, String desc) {
super(name, desc);
}
@Override
public void add(OrganizationComponent organizationComponent) {
this.organizationComponents.add(organizationComponent);
}
@Override
public void remove(OrganizationComponent organizationComponent) {
this.organizationComponents.remove(organizationComponent);
}
@Override
public void print() {
System.out.println("----------" + getName() + "----------");
organizationComponents.forEach(OrganizationComponent::print);
}
}
院系
public class Department extends OrganizationComponent {
public Department(String name, String desc) {
super(name, desc);
}
@Override
public void print() {
System.out.println(getName());
}
}
调用
public class Client {
public static void main(String[] args) {
University university = new University("加州大学洛杉矶分校", "UCLA");
College softwareCollege = new College("软件学院", "Software College");
College informationCollege = new College("信息学院", "Information College");
university.add(softwareCollege);
university.add(informationCollege);
softwareCollege.add(new Department("软件工程","Software Engineering"));
softwareCollege.add(new Department("网络工程","Network Engineering"));
softwareCollege.add(new Department("计算机科学与技术","Computer Science and Technology"));
informationCollege.add(new Department("通信工程", "Communications Engineering"));
informationCollege.add(new Department("信息工程", "Information Engineering"));
university.print();
}
}
源码
Map:Component
HashMap:Composite
Node:Leaf
public interface Map<K,V> {
}
public class HashMap<K,V> extends AbstractMap<K,V> implements Map<K,V>, Cloneable, Serializable {
}
static class Node<K,V> implements Map.Entry<K,V> {
}
Views: 2,835 · Posted: 2019-12-19
————        END        ————
Give me a Star, Thanks:)
https://github.com/fendoudebb/LiteNote扫描下方二维码关注公众号和小程序↓↓↓
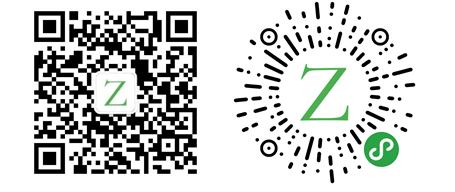
Loading...