设计模式之单例模式
设计模式 Java juc About 1,799 words作用
保证一个类只有一个实例,并提供一个访问它的全局访问点。
饿汉式(静态变量)
- 线程安全
- 未达到懒加载效果,可能造成内存浪费
- @see java.lang.Runtime
public class Singleton1 {
//1. 私有化构造函数,保证外部不能直接new
private Singleton1() {
}
//2. 类内部创建对象实例
private static final Singleton1 instance = new Singleton1();
//3. 提供公有静态方法,返回实例对象
public static Singleton1 getInstance() {
return instance;
}
}
饿汉式(静态代码块)
- 与饿汉式-静态变量效果一样
public class Singleton2 {
private Singleton2() {
}
private static Singleton2 instance;
static {
instance = new Singleton2();
}
public static Singleton2 getInstance() {
return instance;
}
}
懒汉式(同步方法)
- 懒加载、线程安全
- 获取实例时同步,效率降低
public class Singleton3 {
private Singleton3() {
}
private static Singleton3 instance;
//同步代码
public static synchronized Singleton3 getInstance() {
if (instance == null) {
instance = new Singleton3();
}
return instance;
}
}
双重检查
- 懒加载、线程安全
- 效率高
- 推荐使用
public class Singleton4 {
//防止在new对象时指令重排序
private static volatile Singleton4 instance;
private Singleton4() {
}
public static Singleton4 getInstance() {
if (instance == null) {
synchronized (Singleton4.class) {
if (instance == null) {
instance = new Singleton4();
}
}
}
return instance;
}
}
静态内部类
- 懒加载、线程安全
- 当Singleton5加载时,其静态内部类是不会被加载的,只有在调用getInstance()时才加载
- JVM类的装载机制保证线程安全
- 推荐使用
public class Singleton5 {
private Singleton5() {
}
private static class SingleInstance {
private static final Singleton5 INSTANCE = new Singleton5();
}
public static Singleton5 getInstance() {
return SingleInstance.INSTANCE;
}
}
枚举
- 推荐使用
enum Singleton6 {
INSTANCE
}
Views: 2,978 · Posted: 2019-12-11
————        END        ————
Give me a Star, Thanks:)
https://github.com/fendoudebb/LiteNote扫描下方二维码关注公众号和小程序↓↓↓
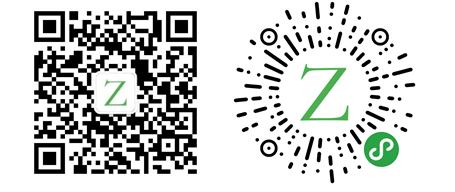
Loading...