Android 使用 libphonenumber 库查询归属地
Android About 7,883 words参考文档
- 开源地址:https://github.com/googlei18n/libphonenumber
- 项目文档:https://javadoc.io/doc/com.googlecode.libphonenumber/libphonenumber/8.5.1
备注
可以三个包重新打包成一个包
jar vcf xxx.jar *
添加依赖
compile 'com.googlecode.libphonenumber:libphonenumber:8.5.1'
compile 'com.googlecode.libphonenumber:carrier:1.62'
compile 'com.googlecode.libphonenumber:geocoder:2.72'
工具类
public class GeoUtil {
private static final String TAG = "zbj0527";
private static PhoneNumberUtil mPhoneNumberUtil = PhoneNumberUtil.getInstance();
private static PhoneNumberToCarrierMapper carrierMapper = PhoneNumberToCarrierMapper.getInstance();
// 获取国家码 “CN”
public static String getCurrentCountryIso(Context context) {
// The {@link CountryDetector} should never return null so this is safe to return as-is.
return CountryDetector.getInstance(context).getCurrentCountryIso();
}
//获取归属地信息
public static String getGeoCodedLocationFor(Context context, String phoneNumber) {
final PhoneNumberOfflineGeocoder geocoder = PhoneNumberOfflineGeocoder.getInstance();
Phonenumber.PhoneNumber structuredNumber = getStructedNumber(context,phoneNumber);
Locale locale = context.getResources().getConfiguration().locale;
return geocoder.getDescriptionForNumber(structuredNumber,locale);
}
//检查是否为 有效号码
public static boolean checkPhoneNumber(Context context,String phoneNumber) {
return mPhoneNumberUtil.isValidNumber(getStructedNumber(context,phoneNumber));
}
public static Phonenumber.PhoneNumber getStructedNumber(Context context,String phoneNumber) {
try {
return mPhoneNumberUtil.parse(phoneNumber,getCurrentCountryIso(context));
} catch (NumberParseException e) {
Log.d(TAG, "getStructedNumber:NumberParseException: " + e);
return null;
}
}
//获取运营商信息
public static String getCarrier(Context context,String phoneNumber) {
Phonenumber.PhoneNumber structedNumber = getStructedNumber(context,phoneNumber);
return carrierMapper.getNameForNumber(structedNumber,Locale.US);
}
}
public class CountryDetector {
private static final String TAG = "zbj0527";
private static final String DEFAULT_COUNTRY_ISO = "CN";
private static CountryDetector sInstance;
private final TelephonyManager mTelephonyManager;
private CountryDetector(Context context) {
mTelephonyManager = (TelephonyManager) context.getSystemService(Context.TELEPHONY_SERVICE);
}
public static CountryDetector getInstance(Context context) {
if(sInstance == null) {
synchronized (CountryDetector.class) {
if (sInstance == null) {
sInstance = new CountryDetector(context);
}
}
}
return sInstance;
}
public String getCurrentCountryIso() {
String result = null;
boolean networkCountryCodeAvailable = isNetworkCountryCodeAvailable();
Log.d(TAG, "networkCountryCodeAvailable: " + networkCountryCodeAvailable);
if (networkCountryCodeAvailable) {
result = getNetworkBasedCountryIso();
Log.d(TAG," getNetworkBasedCountryIso: " + result);
}
if (TextUtils.isEmpty(result)) {
result = getSimBasedCountryIso();
Log.d(TAG,"getSimBasedCountryIso: " + result);
}
if (TextUtils.isEmpty(result)) {
result = getLocaleBasedCountryIso();
Log.d(TAG,"getLocaleBasedCountryIso: " + result);
}
if (TextUtils.isEmpty(result)) {
result = DEFAULT_COUNTRY_ISO;
Log.d(TAG,"DEFAULT_COUNTRY_ISO");
}
Log.d(TAG," result == " + result + "\n-------------------------------------------------");
return result.toUpperCase(Locale.US);
}
/**
* @return the country code of the current telephony network the user is connected to.
*/
private String getNetworkBasedCountryIso() {
return mTelephonyManager.getNetworkCountryIso();
}
/**
* @return the country code of the SIM card currently inserted in the device.
*/
private String getSimBasedCountryIso() {
return mTelephonyManager.getSimCountryIso();
}
/**
* @return the country code of the user's currently selected locale.
*/
private String getLocaleBasedCountryIso() {
Locale defaultLocale = Locale.getDefault();
if (defaultLocale != null) {
return defaultLocale.getCountry();
}
return null;
}
private boolean isNetworkCountryCodeAvailable() {
// On CDMA TelephonyManager.getNetworkCountryIso() just returns the SIM's country code.
// In this case, we want to ignore the value returned and fallback to location instead.
return mTelephonyManager.getPhoneType() == TelephonyManager.PHONE_TYPE_GSM;
}
}
或者
public class PhoneUtil {
private static PhoneNumberUtil phoneNumberUtil = PhoneNumberUtil.getInstance();
private static int countryCode = phoneNumberUtil.getCountryCodeForRegion(Locale.getDefault().getCountry());
private static PhoneNumberToCarrierMapper carrierMapper = PhoneNumberToCarrierMapper.getInstance();
private static PhoneNumberOfflineGeocoder geocoder = PhoneNumberOfflineGeocoder.getInstance();
/**
* 根据国家代码和手机号判断手机号是否有效
*
* @param phoneNumber 手机号
* @param countryCode 国家代码,中国为 86
* @return 手机号是否有效
*/
public static boolean checkPhoneNumber(String phoneNumber, String countryCode){
int ccode = Integer.parseInt(countryCode);
long phone = Long.parseLong(phoneNumber);
Phonenumber.PhoneNumber pn = new Phonenumber.PhoneNumber();
pn.setCountryCode(ccode);
pn.setNationalNumber(phone);
return phoneNumberUtil.isValidNumber(pn);
}
/**
* 根据国家代码和手机号 判断手机运营商
*
* @param phoneNumber 手机号
* @param countryCode 国家代码,中国为 86
* @return 获取手机运营商
*/
public static String getCarrier(String phoneNumber, String countryCode){
int ccode = Integer.parseInt(countryCode);
long phone = Long.parseLong(phoneNumber);
Phonenumber.PhoneNumber pn = new Phonenumber.PhoneNumber();
pn.setCountryCode(ccode);
pn.setNationalNumber(phone);
PhoneNumberUtil.PhoneNumberType numberType = phoneNumberUtil.getNumberType(pn);
//返回结果只有英文,自己转成成中文
String carrierEn = carrierMapper.getNameForNumber(pn, Locale.ENGLISH);
String carrierZh = "";
carrierZh += geocoder.getDescriptionForNumber(pn, Locale.CHINESE);
switch (carrierEn) {
case "China Mobile":
carrierZh += "移动";
break;
case "China Unicom":
carrierZh += "联通";
break;
case "China Telecom":
carrierZh += "电信";
break;
default:
break;
}
carrierZh += numberType.name();
return carrierZh;
}
/**
* 根据手机号获取手机归属地
*
* @param phoneNumber 手机号
* @return 归属地
*/
public static String getGeo(String phoneNumber){
long phone = Long.parseLong(phoneNumber);
Phonenumber.PhoneNumber pn = new Phonenumber.PhoneNumber();
pn.setCountryCode(countryCode);
pn.setNationalNumber(phone);
return geocoder.getDescriptionForNumber(pn, Locale.CHINESE);
}
public static void main(String[] args) {
System.out.println(PhoneUtil.checkPhoneNumber("159655555","86")); // false
System.out.println(PhoneUtil.checkPhoneNumber("18601720000","86")); // true
System.out.println(PhoneUtil.getCarrier("18601720000","86")); // 上海市联通MOBILE
System.out.println(PhoneUtil.getGeo("18601720000")); // 上海市
}
}
Views: 6,709 · Posted: 2019-04-14
————        END        ————
Give me a Star, Thanks:)
https://github.com/fendoudebb/LiteNote扫描下方二维码关注公众号和小程序↓↓↓
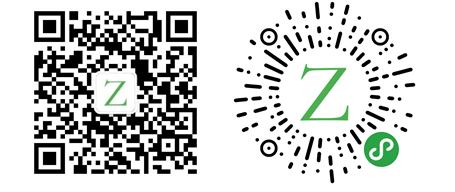
Loading...