Java 消除 if else 代码的几种方式
Java 面试 About 3,473 words提前 return
优化前
if(condition) {
//doSomething
} else {
return;
}
优化后
if(!condition){
return;
}
//doSomething
使用三元运算符
优化前
int price;
if(condition) {
price = 80;
} else {
price = 100;
}
优化后
int price = condition ? 80 : 100;
使用枚举
优化前
int status = 0;
String orderStatus;
if (status == 0) {
orderStatus = "UNPAID";
} else if (status == 1) {
orderStatus = "PAID";
} else {
orderStatus = "PAYING";
}
优化后
public class EnumDemo {
public static void main(String[] args) {
int status = 0;
String orderStatus = OrderStatus.of(status);
}
private enum OrderStatus {
UNPAID(0,"未支付"),
PAID(1,"已支付"),
PAYING(2, "支付中");
private int code;
private String label;
OrderStatus(int code, String label) {
this.code = code;
this.label = label;
}
public static String of(int code) {
for(OrderStatus s : OrderStatus.values()) {
if(s.code == code) {
return s.label;
}
}
return "";
}
}
}
使用 Optional
优化前
String s = "hello world";
if (s != null) {
System.out.println(s);
} else {
System.out.println("str is empty");
}
优化后:Java9
之后Optional
增加的ifPresentOrElse()
方法
String s = "hello world";
Optional.of(s).ifPresentOrElse(System.out::println, () -> System.out.println("empty str"));
表驱动法
优化前
String param = "one";
if ("one".equals(param)) {
System.out.println(param.toUpperCase());
} else if ("two".equals(param)) {
System.out.println(param.toLowerCase());
} else if ("three".equals(param)) {
System.out.println(param.trim());
}
优化后
String param = "one";
HashMap<String, Function<String, String>> map = new HashMap<>();
map.put("one", String::toUpperCase);
map.put("two", String::toLowerCase);
map.put("three", String::trim);
String apply = map.get("three").apply("abc");
System.out.println(apply);
策略模式+工厂方法
优化前
String param = "one";
if ("one".equals(param)) {
System.out.println("this is one");
} else if ("two".equals(param)) {
System.out.println("this is two");
} else if ("three".equals(param)) {
System.out.println("this is three");
}
优化后
public class StrategyAndFactoryDemo {
public static void main(String[] args) {
IStrategy strategy = StrategyFactory.getStrategy("two");
strategy.doSomething();
}
private static class StrategyFactory {
private static final Map<String, IStrategy> map = new HashMap<>();
static {
map.put("one", new StrategyOne());
map.put("two", new StrategyTwo());
map.put("three", new StrategyThree());
}
public static IStrategy getStrategy(String index) {
return map.get(index);
}
}
private interface IStrategy {
void doSomething();
}
private static class StrategyOne implements IStrategy {
@Override
public void doSomething() {
System.out.println("this is strategy one");
}
}
private static class StrategyTwo implements IStrategy {
@Override
public void doSomething() {
System.out.println("this is strategy two");
}
}
private static class StrategyThree implements IStrategy {
@Override
public void doSomething() {
System.out.println("this is strategy three");
}
}
}
Views: 3,853 · Posted: 2021-04-18
————        END        ————
Give me a Star, Thanks:)
https://github.com/fendoudebb/LiteNote扫描下方二维码关注公众号和小程序↓↓↓
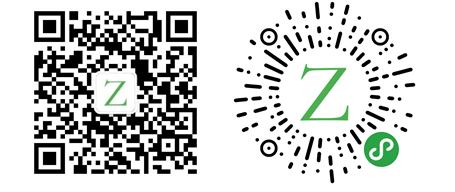
Loading...